Terrain Viewer Documentation
Prepared for CS 526 by John Bell, Spring 2004
Project Background:
This project involved visualizing terrain data, available as
image files. ( One file containing elevation information in a
"gray scale" format, and a separate file containing
imagery suitable for a texture map. ) For further details see
the original project
assignment.
Sample Visualization Output:
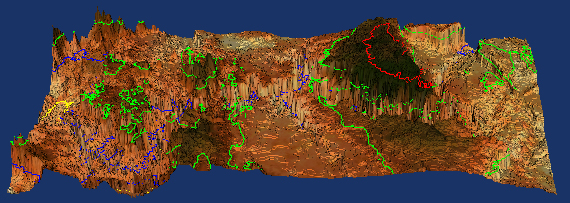
Contours Only ( During Interactive Rotations ):
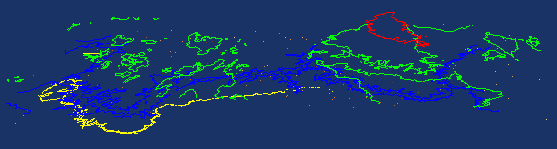
User Interface:
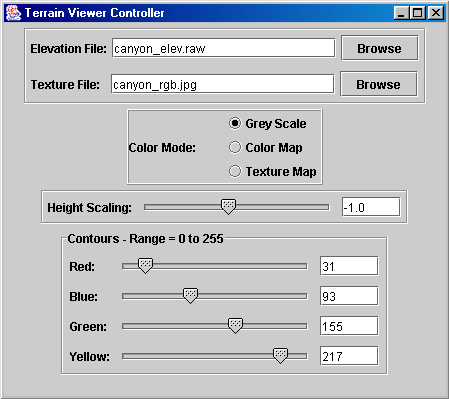
SubTasks Accomplished:
- Set up the VTK environment & successfully developed visualizations
- Read in data from files in either JPG or RAW format
- Created an elevated terrain, based on input data and a specified
scaling factor.
- Displayed the terrain in either grayscale or with an overlaid
texture map.
- Created colored contours at specified data levels, in red,
green, blue, and yellow
- Overlaid the countours on the elevated surface, each at the
correct height.
- Displayed the surface in an interactive fashion
- Created a user interface that allows the user to:
- Enter or select the file from which to read elevation data
- Enter or select the file from which to read texture data
- Select from among gray scale, color mapping, and textured
surface
- Adjust the scaling factor from -10.0 to 10.0
- Adjust the color contour data levels from minimum to maximum
values
- ( The minimum and maximum are queried from the data set,
and updated on file change. )
- For all sliders, users may also type in values within the
valid range, which is checked.
- Integrated a Java based User Interface with a C++ based visualization
model, using the MVC paradigm.
Difficulties Encountered & Possible Future Improvements:
- The biggest difficulty encountered is that the performance
of the interaction between the user interface and the visualization
model is totally unacceptable, and therefore all of the calls
from the user interface to the model have been commented out.
- ( Apparently the problem is that the Model must run in a
separate Thread in order to have two windows open simultaneously,
but the Singleton pattern is not functioning properly across
multiple threads, and therefore the system is trying to create
and run two separate instances of the Model whenever messages
are sent from the UI to the Model. )
- The code is written to handle arbitrary input files. However
due to the difficulty mentioned above, the provision to select
an alternate input file has been disabled, and hence no second
data set has been implemented.
- The colormapping option has not been implemented.
- The code has not been tested at EVL.
-
- The code needs improved documentation ( commenting ), and
some cleaning up. ( E.g. the separate JSlider objects "Red",
"Green", "Blue", "Yellow", and
"Scale" should be placed in an array instead of being
handled individually. This is actually done in the Model portion
of the code, but not in the View portion. )
- It would be nice to implement a menu bar with pull-down help,
plus context-sensitive help such as tool tips.
- Flexibility of the contours would allow the user to select
an arbitrary number and assign arbitrary colors.
- Data picking would allow the user to point to a point on
the data surface and query the local value.
System Architecture and Major Source Code Files
The system is designed along the Model-View-Controller Paradigm,
with the following key files in each portion. Note that for the
Java code, there are also associated C++ files to implement the
Java-Native Interface. These files essentially contain methods
that are members of the Java classes, but which are written in
C++, and which are therefore able to call on other C++ class methods.
( E.g. so the Controller and View written in Java can send messages
to the Model written in C++. )
Controller:
- The controller starts the program, and creates instances
of the Model and View classes.
- TerrainViewer.java is the
main code file for this class.
- TerrainViewer.cpp contains
the C++ native code implemented as part of the TerrainViewer
class.
- TerrainViewer.h contains the
function prototypes for TerrainViewer.cpp
- Class files generated when TerrainViewer.java is compiled
are TerrainViewer.class, and
windowCloser.class
- Note that the Model is created in a separate Java Thread
( in the ModelLauncher class ), in order to invoke multiple windows
simultaneously. The java code is included in TerrainViewer.java,
and the C++ source files associated with the ModelLauncher class
are ModelLauncher.h, and ModelLauncher.cpp;
The resulting class file is ModelLauncher.class.
Model:
- The model incorporates all of the VTK classes and data visualization
pipeline.
- TerrainViewerModel.cxx
is the code file for this class. The prototypes are defined in
TerrainViewerModel.h.
- The model responds to messages sent from the user interface,
and adjusts the visualization pipeline accordingly.
- Note that the model is implemented using the Singleton pattern
- The constructor is private, and the static method getModel(
) ensures that only one instance is ever instantiated, the first
time it is needed.
View:
- The view is the user interface, written primarily in Java,
with native interface code to access the model
- TerrainViewerUI.java is
the main code file for this class.
- TerrainViewerUI.cpp contains
the C++ native code implemented as part of the TerrainViewerUI
class. The corresponding header file is TerrainViewerUI.h.
- Utils.java, ImageFilter.java,
and RawFilter.java are small support
files for the UI. The first two were downloaded from Sun's Java
Tutorial pages, and the last is a simple modification of the
second. The class files generated by these source files are Utils.class, ImageFilter.class,
and RawFilter.class respectively.
- The user interface performs basic error checking of user
input, and then sends messages to the model whenver any input
values change.
Building and Running the Application
Building and running this application requires three main steps:
- Compile and link all of the C++ files, and store the results
in a DLL file. The Microsoft Studio files TerrainViewerModel.dsw
and TerrainViewerModel.dsp
should provide this functionality with the "build"
button, which should generate the file TerrainViewerModel.dll.
- Compile the Java modules, which requires the following commands
from the command line:
- set CLASSPATH=.
- javac TerrainViewer.java
- javac TerrainViewerUI.java
- Run the program from the command line: